Remove Matching Object From JavaScript Array
Functions used in this tutorial:
- array.some – check if at least one item exists in array.
- array.findIndex – find index of an object in array of objects.
- array.splice(index, howManyToDelete) – split array at index and remove n-number of items past that point, return an item or list of items that were removed.
Sometimes you need to remove an object from an array that contains properties that match a value (or values.) This tutorial explores one practical use case for this.
What is a practical use case for removing objects from JavaScript array?
One of those use cases is storing association between user and event. For example, when user "likes" a tweet, we store relationship between user id and tweet id. This entry is stored in Mongo (or any other) database. When user "unlikes" the tweet, we need to remove that entry from Array object in event relationship map.
In my case I had to remove entry from array when developing Semicolon (this site!) Here's the example of removing object from JavaScript array and what it was used for:
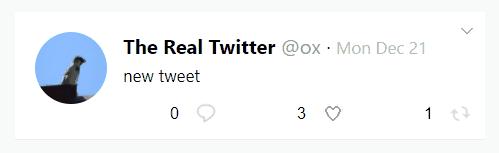
There are many ways of removing an object from a JavaScript array. This tutorial shows how to remove an object with two properties. The removal of the object will occur only if it matches two values provided in a filter object.
let relationships = [ { userid: 1, tweetid: 2 }, // user 1 likes tweet 2 { userid: 2, tweetid: 2 }, // user 2 likes tweet 2 { userid: 3, tweetid: 4 }, // user 3 likes tweet 4 ]
Let's say we want to remove 2nd item:
let filter = { userid: 2, tweetid: 2 }
Basically filter is an object that works as a filter for the item we want to delete. It is not stored as a reference to that object. It's a separate, stand-alone variable.
I wrote this helper equals function because I need to match two properties in an object. If in your case you need to test only one property, you can remove the second test after && operator or provide alternative matching criteria you're looking for.
// Utility function for comparing a relationship // between user id and tweet id const equals = (list, filter) => list.some( v => v.userid === filter.userid && v.tweetid === filter.tweetid );
I used the built-in .some method, because it will break out of the loop, soon as at least one item is found – exactly what we need – without spending any more time parsing entire array, like some of the other methods do (like filter or map, for example.) Doing this might help with performance on large lists.
The equals function takes the array containing all relationship associations in its first argument. The second argument is the filter object. It's an object containing properties we want to match and then delete an entry that matches it from the list.
Now we just need to splice the array
The [].splice(index, howmany) method deletes an item or a number of items from the array starting from index (also deleting item at index.)
If you don't provide second argument splice will remove all items after index specified in first argument. This is why we will use splice(array, 1), because we only need to remove one item.
In order to splice an array we need to know the index position of the item in the array we want to start splicing it. (Or deleting one or more items starting at that location.)
First, let's find that index using the build-in Array method findIndex:
// filter object containing properties that match // property values of the array item we want to remove let filter = { userid: 2, tweetid: 2 } // first we need to find index of the object // we're looking to remove: let index = relationships.findIndex( i => i.userid === remove.userid && i.tweetid === remove.tweetid); // finally, remove matched item relationships.splice(index, 1)
One traditional way of removing an item from JavaScript array is by using the built-in splice method. And that's what we did here.
Last argument is set to 1 to make sure we only delete 1 item.
You can specify any number here, but in this case we only need to delete 1.
Leaving second argument empty will remove all items after the index.
This tutorial came about when I needed to add and remove the relationship between a user id and the tweet object, indicating that it was "liked" by a particular user.
Clicking the "like" button multiple times would toggle the object, inserting it or removing it from live relationship object.
By sharing it with others, I hope it helps someone out there who is facing a similar issue. Inevitably, you'll come across something like this in your own code.