Difference Between ...spread and ...rest In JavaScript
In JavaScript both rest the spread operators are represented by the 3 dots ...
The spread operator is used to distribute all of its items into another array or object. It can be used to merge multiple arrays, merge all values of one array into another array, or merge properties of multiple objects into one object, including JavaScript sets.
The rest operator (referred to as rest parameters) converts a list of values into an array. It can be passed into a function as a regular parameter list, separated by comma. Inside the function, that list of variables will be available as an array of those values.
spread looks exactly like rest operator, this is why it's important to know the difference.
What is the difference between rest and spread operators in JavaScript?
The primary difference between spread and rest is that the rest operation puts "the rest of" primitive values (1, "a",etc) into the new object or array. This is why often, rest operator is used as the last item on a total list of values. As "the rest of the values." But spread syntax takes iterable objects (arrays, sets or objects with properties,) converts them to a list of individual values, and combines them with values or properties that already exist in another object of same kind.
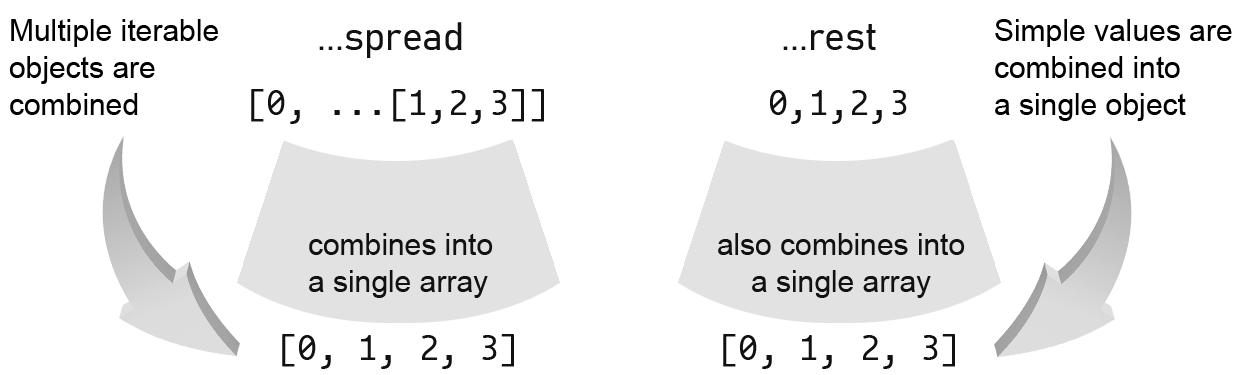
The difference between spread and rest is not as much in the result of the operation.
They both combine result into an array, set or an object.
They differ in which data type is used and how data is passed to those operators.
Here are two classic use-cases for rest operator:
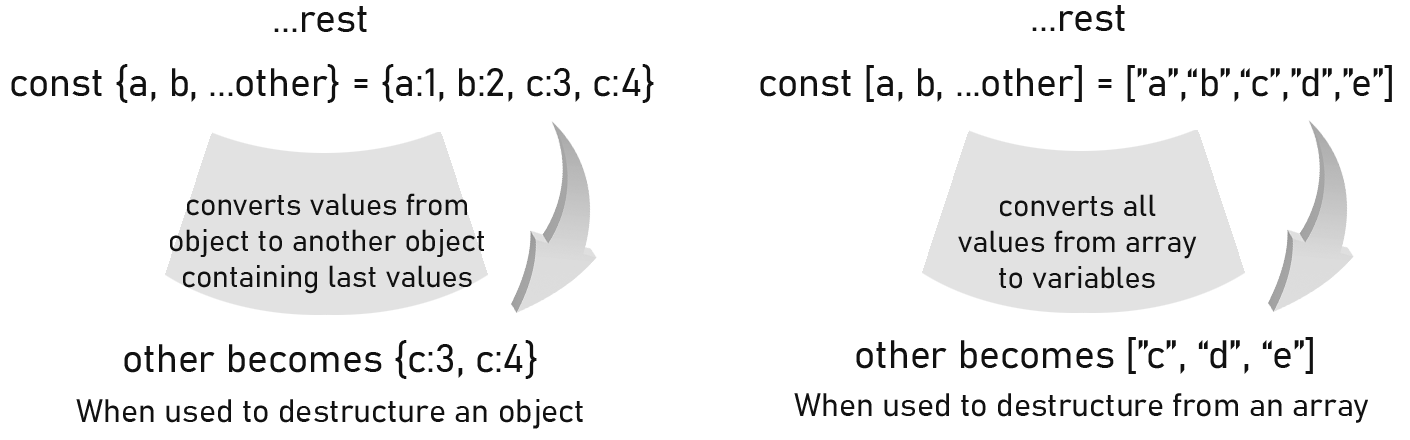
When destructuring from array:
const [a, b, ...other] = [1,2,3,4,5]
Here ...other will break into a separate array containing values [3,4,5].
const {a, ...other} = {a:1, b:2, c:3}
And in the above example ...other will hold {b:2, c:3}.
As you can see it's literally "the rest of" values.
Other examples of rest and spread are shown below.
How to use ...rest operator to specify unknown number of function parameters
The rest operator is also referred to as rest parameters.
Think of rest as "the rest of values", which can be represented by one or many more values.
With rest parameters, number of values is ambiguous on purpose, because it assumes one or more values. This makes rest operator perfect for being used for passing an unknown number of arguments to a function. But only when it's last value on the argument list.
When using rest as function parameters, it should always be last parameter on the list (Because if it is followed by any more single arguments, it'll be confusing as to how values should be mapped, because rest can represent an array of one, or more values.)
Here is an example of rest operator used to define unknown number of additional function parameters:
function printFruit(first, second, ...others) { console.log(first); console.log(second); console.log(others); } printFruit("Apple", "Banana", "Orange", "Pineapple", "Strawberry")
And the result of this example is printed below:
AppleBanana
["Orange", "Pineapple", "Strawberry"]
Here "Apple" and "Banana" printed as individual function arguments.
But "Orange", "Pineapple" and "Strawberry" were received inside the function as an array fetched from ...others argument. Here rest operator packed "indefinite" number of arguments into a single array.
Notice that it's important for rest operator to be last on the list of parameters. Placing it prior any other parameters on the list is not going to work.
Difference between ...rest parameters and arguments object inside a function
As you may know, JavaScript functions automatically have access to a variable named arguments inside the body of that function.
Because rest can be used to build function parameters, let's mention the differences between rest and the array-lie arguments object.
Difference 1: The arguments object is an array-like object, it isn't a real array
The arguments object contains a list of all values passed into the function on its argument list. Oddly, this array-like arguments object only appears to be an array of items. But it's not a real JavaScript array.
Meanwhile, the rest operator will produce a real, iterable array when used in a function parameter list.
This is why you should prefer rest parameters over the arguments arrays. Simply because iterable arrays are much easier to work with.
Difference 2: The arguments object cannot be used in an arrow function
One limitation of arguments object is that it can only be used inside a regular JavaScript function that was defined using the function keyword.
Arguments array is not available inside arrow functions.
Difference 3: Use rest over arguments object and heed to ES6 standards
The rest operator is a more standard way of writing ES6-compliant JavaScript code.
Again, another reason to use rest operator instead, for uniformity and best practice.
How is spread operator different from rest?
You can somewhat think of spread operator as opposite of rest.
It unpacks values from an array (or object) into individual variables.
Spread operator always precedes an object or an array:
console.log(...[1,2,3])
Will print:
1 2 3
You cannot use ... operator when assigning its value to a single variable:
const a = ...[1,2,3]
Code above will produce an error.
But you can freely use it as a parameter list as shown in next example.
Here is a function that takes 3 arguments. You can pass it an array of values using spread operator. These values will automatically "map" to the function arguments.
function printFruit(first, second, third) { console.log(first) console.log(second) console.log(third) }
Now let's pass an array of values into this function using spread operator:
// Call the function: printFruit(...["Orange", "Strawberry", "Banana"]);
In a way, we are destructuring array of values into function parameters.
And the result printed by console will be:
OrangeStrawberry
Banana
The spread operator was used to unpack an array of arguments into function parameters.
In the same way, spread can be used inside an array or an object as part of its value set.
How to use ...spread operator to combine two or more arrays
First, here is a spread operator example used to combine or "spread" values of another array into the parent array:
let array = [1, ...[5,7]]; console.log(array)
Here 5 and 7 are the values that were spread into another array.
Result:
[1, 5, 7]
Here the spread operator was used to unroll elements in an array into another array, which already contained another item.
Here is an example of spread operator used to spread items of two arrays into a single array:
let array = [...[0, 1, 2, 3], ...[5, 7]]; console.log(array)
The result of this code will be:
[0, 1, 2, 3, 5, 7]
How to use ...spread operator to combine object properties into one object
In the same way you can combine multiple objects into one using spread operation:
const one = {a:1, b:2, c:3}; const two = {d:4, e:5}; console.log({...one, ...two});
Result:
{ a:1, b:2, c:3, d:4, e:5 }
Keywords you might be using to find this article on search engines:
- js spread operator merge array
- javascript spread operator uses
- js spread operator reference
- js spread operator order
Conclusion
Use rest and spread operators when you need to unpack a list of values into a function or another array or object.