Create a New Blank React App with TypeScript
In this brief tutorial we'll go over the steps required to create a new React app with TypeScript support (Make sure to install NodeJS, so we have access to npx)
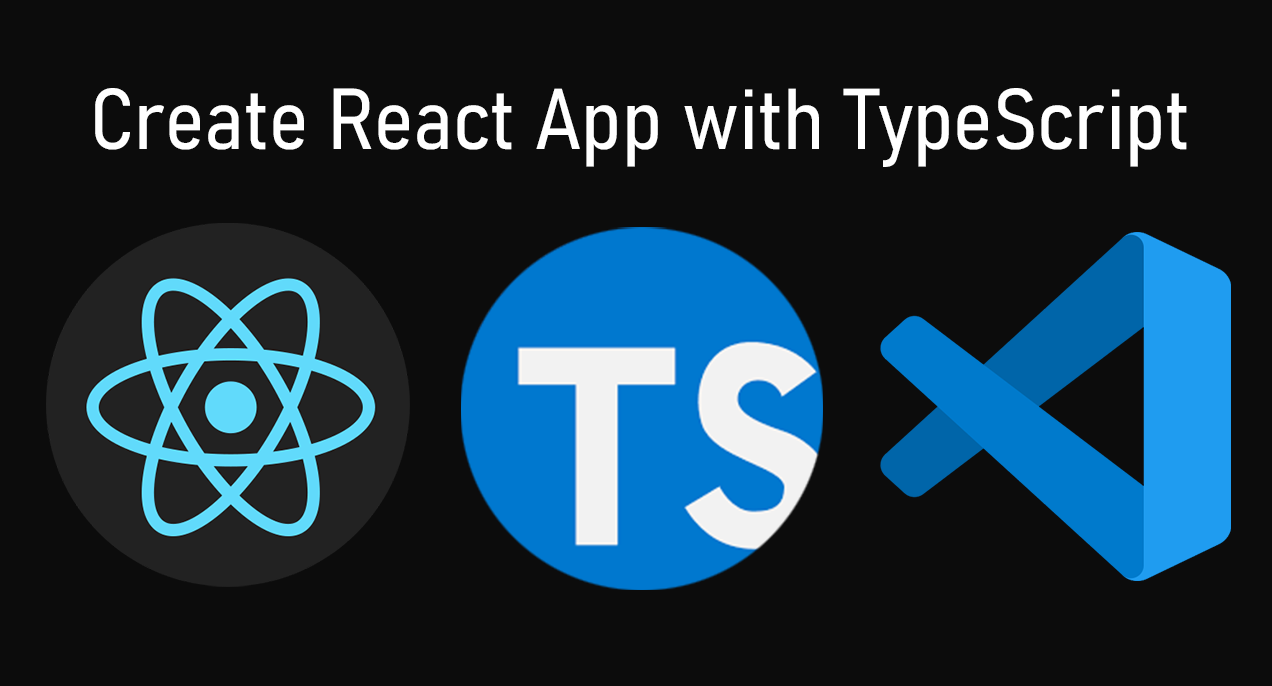
Learn how to create a new React app with TypeScript support preinstalled
To create a new React app with TypeScript support, follow these steps:
- Download (here) and Install NodeJS on your system (this will give us npx command)
- Create a new folder, and go there in your Terminal, Bash or cmd.exe
Make sure you have NodeJS installed and check it from command line:
node --version
Also:
npx --version
Both of the commands above should print out Node and NPX version currently installed on your computer. If you get errors, you need to install NodeJS first.
To create a new React app with TypeScript type this in Terminal:
npx create-react-app myProject --template typescript
If for some reason you get an error, alternatively you can try forcing for latest version:
npx create-react-app@latest myProject --template typescript
Adding --template typescript is the key to making React with TypeScript apps from scratch.
Next make sure to install TypeScript dependencies.
In Terminal run the following npm command:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
Or if you're using yarn type:
yarn add typescript @types/node @types/react @types/react-dom @types/jest
Remember to rename your .js files to .ts, where you want to use TypeScript. But if you are also using JSX with React, make sure to rename those files to .tsx.
Locate your TypeScript configuration file tsconfig.json (you'll need it later)
Where is tsconfig.json in your React project? It's in the project root folder!
TypeScript configuration is stored in tsconfig.json file at the root of your project (same folder where your package.json file is). And here's an example with default settings:
{ "compilerOptions": { "target": "es6", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "forceConsistentCasingInFileNames": true, "noFallthroughCasesInSwitch": true, "module": "esnext", "moduleResolution": "node", "resolveJsonModule": true, "isolatedModules": true, "noEmit": true, "jsx": "react-jsx" }, "include": ["src//*"] }
Here two of the most important settings are probably: strict: true and target: "es6".
By default TypeScript installation will adhere to ES6 ruleset, which is things like async/await, arrow functions, for...of loops, const, let, and all those common JavaScript features.
You can turn strict mode on or off, just pass false to strict property if you need to turn it off for some reason.
I made this video demonstrating an example of building a React app with Typescript:
So now that you installed TypeScript in your React App, how does TypeScript work?
How To Create Types In TypeScript
Define a type, let's say, Trick (as in magic trick):
type Trick = {
name: string,
power: number,
}
In TypeScript this is just a definition of a new unique type.
Note that there are no actual values defined, just their names and types.
A magic trick type Trick has a name (string) and power level (number).
Maybe spell casting could be used for a Role-Playing game you're developing, and you need to know how much damage a magic trick causes to your enemies?
It can be anything you want based on the problem you're trying to solve, just use your creativity.
One quick tip for creating types in TypeScript
The key is to define a composition of different types making up this object.
Don't overdesign types by trying to define as many things as possible. Think about the absolute minimum number of properties and their types the object you're trying to define should have.
A car could have wheels (number), color (string) and number of doors (number), for example.
Keep things as simple as possible.
What is the purpose of using TypeScript?
Creating types in TypeScript can make your code safer.
It prevents you from assigning a value that doesn't match the type defined by the custom pattern you create by using the type keyword (as we've seen earlier with magic trick!).
But what is code safety?
Code safety is a way to limit type-based mistakes in your code to a minimum.
Think of TypeScript as a safeguard.
If assigned values don't agree with the types of an object they should take on a form of, TypeScript will give you a compilation error:
let magictrick:Trick = 100;
In this example, TypeScript gave us an error.
We can't assign just about any value to a variable of type Trick.
Here we assigned the value 100 which is a number to magictrick which is of type Trick.
In this case value must be an object with exactly two properties: name and power.
And both of them must have a value matching the type defined in Trick type. If this type safety test fails, your program will produce an exception error and stop running.
And that's a good thing. If we fix this error, you ensure that your code will not run into potential errors later on, caused by assigning objects to variables of a type they were never intended to have.
To prevent TypeScript errors from happening, make sure to assign values defined with :Trick type to an actual object representing that type:
let magictrick:Trick = {
name: "tidalwave",
power: 75,
};
Now our program will compile without errors.
How to add TypeScript to an existing React app
Here's another video explaining how to add TypeScript type checking to a React app (that was created using create-react-app,) AKA to an existing React app: